Map Generation Tutorial Part 2: Fall Off Map
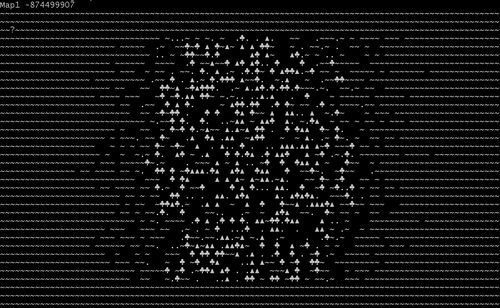
Welcome back to the second part of the Map Generation tutorial! In the previous tutorial, I generated a random ASCII map using Perlin noise, but I'm not satisfied with the result, I wanted to create one main land mass, like a continent or an island. In this tutorial, I will show you one way to achieve this using fall off maps.
A fall off map is a type of map that assigns varying values or intensities to different areas of a given space, with the intensity typically decreasing as the distance from a particular point increases. Fall off maps can be used for a variety of purposes, including creating natural-looking lighting effects, generating terrain features, or simulating atmospheric conditions.
Let's dive into the code. In our map script from part 1, we need to add variables for the center x, center y, and fall off map to our global variable definitions:
private readonly int centerX; private readonly int centerY; double[,] falloffMap;
We then assign these variables in the constructor based on the width and height of the map:
centerX = mapWidth / 2; centerY = mapHeight / 2; falloffMap = new double[mapWidth, mapHeight];
Next, we add the GenerateFalloffMap()
method, which generates our fall off map:
public void GenerateFalloffMap() { for (int y = 0; y < mapHeight; y++) { for (int x = 0; x < mapWidth; x++) { double distance = DistanceFromCenter(x, y); distance = distance / Math.Max(centerX, centerY); falloffMap[x, y] = Math.Max(0, 1 - distance * distance); } } }
We also need to add the DistanceFromCenter()
method to calculate the distance of a pixel from the center point, which is used in the GenerateFalloffMap()
method:
private double DistanceFromCenter(double x, double y) { double deltaX = x - centerX; double deltaY = y - centerY; return Math.Sqrt(deltaX * deltaX + deltaY * deltaY); }
Finally, we combine the fall off map with the Perlin noise map by subtracting the value of the fall off map from the value of the Perlin noise map for each element. When we assign this combined value to our map, we are able to get the land masses we envisioned for this project:
double noise = PerlinNoise(x, y); double falloff = falloffMap[x, y]; double combinedValue = noise - falloff; if (combinedValue > -.8) map[x, y] = Program.waterTile; else if (noise > .066) map[x, y] = Program.mountainTile; else if (noise > 0) map[x, y] = Program.forestTile; else if (noise > -.05) map[x, y] = Program.swampTile; else map[x, y] = Program.plainsTile;
And that's it! We have generated a fall off map in C# and used it to create land masses in our map generator. Check out the supplied image to see the result.
Thank you for reading, and I hope you found this tutorial helpful.
Endless Prose
The long awaited 3rd addition to the Epic Prose series!
Status | In development |
Author | logicandchaos |
Genre | Role Playing |
Tags | Text based |
More posts
- Finishing the LibraryApr 05, 2024
- Text Adventure Library - Builder PatternMar 27, 2024
- Text Adventure Library - Slaying the Spaghetti Monster!Nov 22, 2023
- From Prototype to ProductionSep 25, 2023
- Redesign!Jul 22, 2023
- Improving the Name Generator using ChatGPTApr 20, 2023
- Creatures & Encounters!Mar 30, 2023
- Travelling Across The MapMar 23, 2023
- Exploring DungeonsMar 13, 2023
Leave a comment
Log in with itch.io to leave a comment.