Fog Of War!
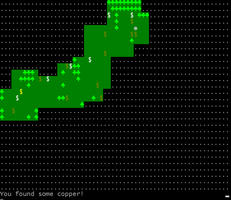
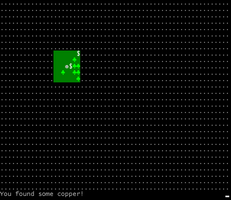
Hello! In this tutorial, I'll be exploring how to create a Fog of War mechanic to conceal your game map. Previously, I showed you how to use a random walker algorithm to create dungeons. This time, we'll be using a 2D array of bools to create a Fog of War. First we need to define the array:
bool[,] fog;
We'll make a CreateFogOfWar()
method and initialize the array to the size of the dungeon map, then, we'll set all tiles on the game map to be covered by fog by default using a nested for
loop:
void CreateFogOfWar() { fog = new bool[width, height]; for (int y = 0; y < height; y++) { for (int x = 0; x < width; x++) { fog[x, y] = true; } } }
This sets each element of the Fog of War array to true
, indicating that all tiles on the game map are initially covered by fog.
Next, we'll need to determine the area around the player that should be uncovered by the Fog of War. To do this, we'll use another nested for
loop, this is done in the print method to uncover the dungeon as you move, I added new parameters for player position and light intensity which is how much of the map to uncover around the player:
public void Print(int playerX, int playerY, int lightIntensity) { for (int y = playerY - lightIntensity; y <= playerY + lightIntensity; y++) { for (int x = playerX - (int)(lightIntensity * 1.5); x <= playerX + (int)(lightIntensity * 1.5); x++) { if (x >= 0 && x < map.GetLength(0) && y >= 0 && y < map.GetLength(1)) { fog[x, y] = false; } } } ..
This loop iterates through all tiles within a certain distance of the player's position and uncovers them by setting their corresponding element in the Fog of War array to false
. The lightIntensity
parameter determines the radius of the uncovered area around the player, while the (int)(lightIntensity * 1.5)
expression determines the width of the uncovered area to compensate for the fact that tiles are taller than they are wide.
Finally, we'll loop through all tiles on the game map and print them based on whether they are uncovered by the Fog of War:
.. for (int y = 0; y < map.GetLength(1); y++) { for (int x = 0; x < map.GetLength(0); x++) { if (!fog[x, y]) { // Print the tile if it is uncovered by the fog of war if (x == playerX && y == playerY) playerTile.Print(); else map[x, y].Print(); } else { // Print the fog of war tile if the tile is covered by fog fowTile.Print(); } } Console.WriteLine(); } }
This loop goes through each tile on the game map and prints it to the console based on whether it is uncovered by the Fog of War. If the tile is uncovered, then it is printed using the appropriate tile's Print()
method. If the tile is covered by the Fog of War, then the fowTile
is printed instead.
That's it! With these steps, you should now be able to print the game map with the Fog of War mechanic applied. Now if you wanted to create a dark dungeon that stays dark except around the player, you can just recover the fog of war at the top of the Print method. Result image included as usual. Good luck with your game development!
Endless Prose
The long awaited 3rd addition to the Epic Prose series!
Status | In development |
Author | logicandchaos |
Genre | Role Playing |
Tags | Text based |
More posts
- Finishing the LibraryApr 05, 2024
- Text Adventure Library - Builder PatternMar 27, 2024
- Text Adventure Library - Slaying the Spaghetti Monster!Nov 22, 2023
- From Prototype to ProductionSep 25, 2023
- Redesign!Jul 22, 2023
- Improving the Name Generator using ChatGPTApr 20, 2023
- Creatures & Encounters!Mar 30, 2023
- Travelling Across The MapMar 23, 2023
- Exploring DungeonsMar 13, 2023
Leave a comment
Log in with itch.io to leave a comment.